データの更新
13.5 データの更新
本節では、WebアプリケーションからJDBCを利用し、MySQLデータベースに登録されたデータを更新する方法について学習します。
データを更新するプログラム
入力フォームから更新対象のIDと更新後の情報を入力し、入力された情報をもとにMySQLデータベースに登録されたデータを更新します。
実行結果
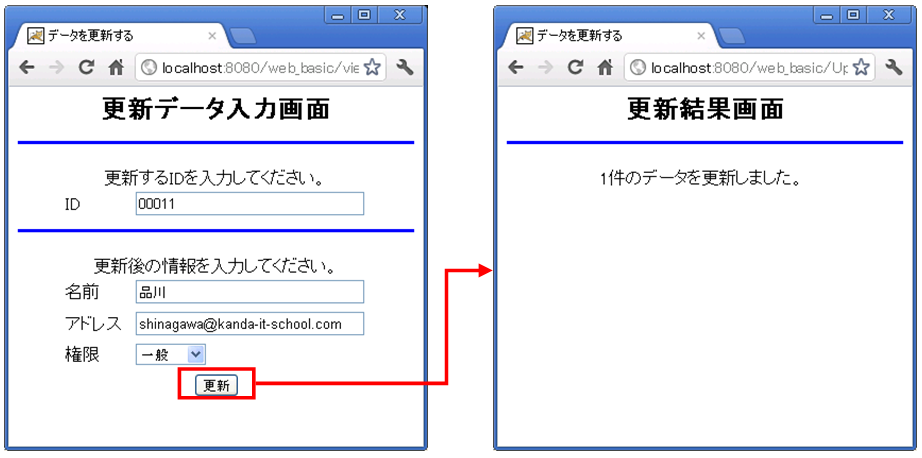
アプリケーション構成
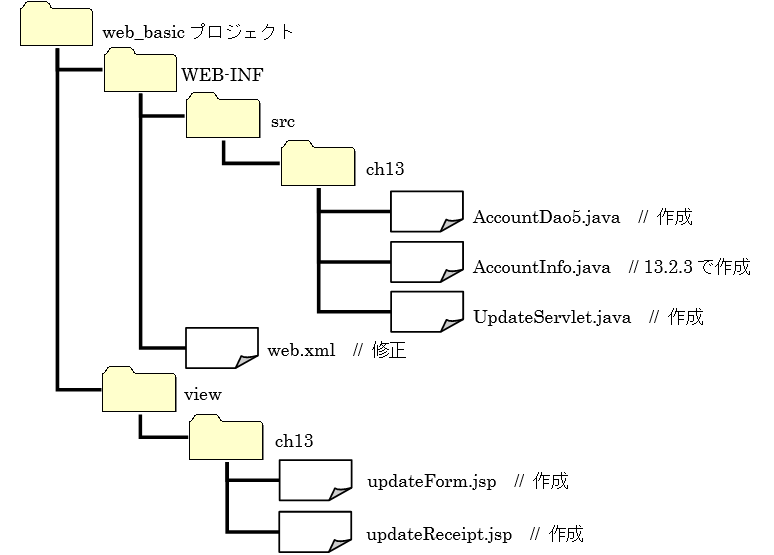
① ソース・フォルダ :web_basic/WEB-INF/src
② パッケージ :ch13
③ 名前 :AccountDao5
➢ AccountDao5.java
5 | public class AccountDao5 { |
8 | private static String RDB_DRIVE = "com.mysql.jdbc.Driver"; |
9 | private static String URL = "jdbc:mysql://localhost/accountdb"; |
10 | private static String USER = "root"; |
11 | private static String PASS = "root123"; |
14 | public static Connection getConnection(){ |
16 | Class.forName(RDB_DRIVE); |
17 | Connection con = DriverManager.getConnection(URL, USER, PASS); |
20 | throw new IllegalStateException(e); |
25 | public int update(AccountInfo accountinfo){ |
27 | Connection con = null; |
34 | String sql = "UPDATE account SET " |
35 | + "name = '" + accountinfo.getName() + "', " |
36 | + "email = '" + accountinfo.getEmail() + "', " |
37 | + "authority = '" + accountinfo.getAuthority() + "' " |
38 | + "WHERE id = '" + accountinfo.getId() + "'"; |
41 | con = getConnection(); |
42 | smt = con.createStatement(); |
45 | count = smt.executeUpdate(sql); |
48 | throw new IllegalStateException(e); |
52 | try{smt.close();}catch(SQLException ignore){} |
55 | try{con.close();}catch(SQLException ignore){} |
① 親フォルダの入力または選択 :web_basic/view/ch13
② ファイル名 :updateForm.jsp
③ アクセスURL :http://localhost:8080/web_basic/view/ch13/updateForm.jsp
➢ updateForm.jsp
1 | <%@page contentType="text/html; charset=UTF-8"%> |
5 | < title >データを更新する</ title > |
8 | < div style = "text-align:center" > |
9 | < h2 style = "text-align:center" >更新データ入力画面</ h2 > |
10 | < hr style = "height:3; background-color:#0000ff" /> |
12 | < form action="<%=request.getContextPath() %>/UpdateServlet"> |
14 | < table style = "margin:0 auto" > |
16 | < td style = "width:60" >ID</ td > |
17 | < td >< input type = text size = "30" name = "id" ></ input ></ td > |
20 | < hr style = "height:3; background-color:#0000ff" /> |
23 | < table style = "margin:0 auto" > |
25 | < td style = "width:60" >名前</ td > |
26 | < td >< input type = text size = "30" name = "name" ></ input ></ td > |
29 | < td style = "width:70" >アドレス</ td > |
30 | < td >< input type = text size = "30" name = "email" ></ input ></ td > |
33 | < td style = "width:60" >権限</ td > |
35 | < select name = "authority" > |
36 | < option value = "管理者" >管理者</ option > |
37 | < option value = "一般" >一般</ option > |
42 | < td colspan = 2 style = "text-align:center" > |
43 | < input type = "submit" value = "更新" > |
① ソース・フォルダ :web_basic/WEB-INF/src
② パッケージ :ch13
③ 名前 :UpdateServlet
④ スーパークラス :javax.servlet.http.HttpServlet
⑤ アクセスURL :updateForm.jspからの画面遷移でアクセスされる
➢ UpdateServlet.java
5 | import javax.servlet.http.*; |
7 | public class UpdateServlet extends HttpServlet{ |
9 | public void doGet(HttpServletRequest request ,HttpServletResponse response) |
10 | throws ServletException ,IOException{ |
16 | request.setCharacterEncoding("UTF-8"); |
19 | AccountInfo accountinfo = new AccountInfo(); |
22 | accountinfo.setId(request.getParameter("id")); |
23 | accountinfo.setName(request.getParameter("name")); |
24 | accountinfo.setEmail(request.getParameter("email")); |
25 | accountinfo.setAuthority(request.getParameter("authority")); |
28 | AccountDao5 objDao5 = new AccountDao5(); |
31 | int count = objDao5.update(accountinfo); |
34 | request.setAttribute("count", count); |
36 | }catch (IllegalStateException e) { |
37 | error ="DB接続エラーの為、更新できませんでした。"; |
40 | error ="予期せぬエラーが発生しました。< br >"+e; |
43 | request.setAttribute("error", error); |
44 | request.getRequestDispatcher("/view/ch13/updateReceipt.jsp").forward(request, response); |
➢ web.xml
2 | < servlet-name >UpdateServletMapping</ servlet-name > |
3 | < servlet-class >ch13.UpdateServlet</ servlet-class > |
6 | < servlet-name >UpdateServletMapping</ servlet-name > |
7 | < url-pattern >/UpdateServlet</ url-pattern > |
① 親フォルダの入力または選択 :web_basic/view/ch13
② ファイル名 :updateReceipt.jsp
③ アクセスURL :UpdateServlet.javaからの画面遷移でアクセスされる
➢ updateReceipt.jsp
1 | <%@page contentType="text/html; charset=UTF-8"%> |
4 | Integer count = (Integer)request.getAttribute("count"); |
5 | String error = (String)request.getAttribute("error"); |
10 | < title >データを更新する</ title > |
13 | < div style = "text-align:center" > |
14 | < h2 style = "text-align:center" >更新結果画面</ h2 > |
15 | < hr style = "height:3; background-color:#0000ff" /> |
18 | <% if(count != null){ %> |
19 | <%= count%>件のデータを更新しました。 |
解説
今回のプログラムは、前節で作成したデータを登録するプログラムとほぼ同じ動きを行っています。異なるのは、情報を入力する画面と表示する画面のレイアウトと、DAOクラスに定義されたメソッドの2点です。
DAOクラスでは、引数に受け取った情報をもとにデータを更新するupdate()メソッドが定義されています。このupdate()メソッドをUpdateServlet.javaの31行目で利用することによって、データベースに登録されたデータの更新を行っています。
31:int count = objDao5.update(accountinfo);
update ()メソッドもinsert()メソッド同様に更新件数が戻り値として定義されており、戻り値として受け取った更新件数を34行目でリクエストスコープへ登録しています。
34:request.setAttribute("count", count);
登録された更新件数はupdateReceipt.jspで取得され、画面へ表示されます。
19:<%= count%>件のデータを更新しました。
データを登録するプログラムと同様に、このプログラムでも更新された件数のみが表示されます。データが正しく更新されたかどうかを確認する場合は、13.3.1項で作成した一覧を表示するプログラムを実行してください。
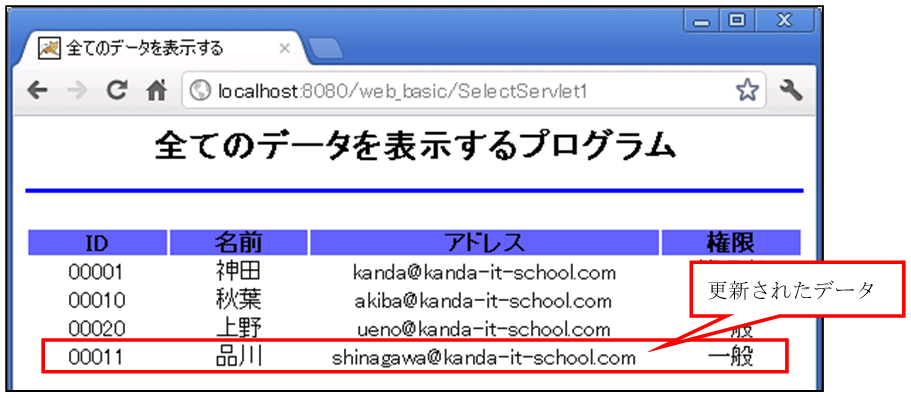
図 13.6.1 データ更新後の一覧表示
次の節では簡単なデータを削除するWebアプリケーションを作成し、データの更新方法を学習します。
NEXT>> 13.6 データの削除